Blockchain DAOs are transforming charitable funds by introducing transparency, decentralization, and community governance to the donation process. Instead of relying on traditional centralized entities to manage funds, DAOs use smart contracts to automate the allocation and distribution of donations.
This ensures that funds are used efficiently and transparently, with the community having a say in how the funds are allocated. The UkraineDAO is a notable example, where donors can vote on funding proposals and track how their donations are being used in real-time. Overall, blockchain DAOs are revolutionizing charitable giving by making it more transparent, efficient, and community-driven.
Process of it
The process of how blockchain DAOs transform charitable funds typically involves several key steps.
- Creation of the DAO: A group of individuals or organizations create a DAO on a blockchain platform. They define the mission, goals, and governance structure of the DAO, including how funds will be managed and distributed.
- Tokenization of Funds: Charitable funds are tokenized, meaning they are converted into digital tokens on the blockchain. Each token represents a share or portion of the total funds.
- Community Participation: Anyone can participate in the DAO by acquiring tokens. This gives them a stake in the organization and the ability to participate in governance decisions, such as approving funding proposals.
- Proposal Submission: Individuals or organizations can submit proposals for funding, outlining how they plan to use the funds for charitable purposes.
- Voting: Token holders vote on funding proposals. The voting process is typically transparent and conducted on-chain, meaning that the results are publicly accessible and tamper-proof.
- Funding Allocation: Approved proposals receive funding in the form of tokens. The DAO's smart contract automatically executes the transfer of tokens to the recipient's address.
- Transparency and Accountability: Since all transactions and decisions are recorded on the blockchain, there is a high level of transparency and accountability. Donors can track how their donations are being used in real-time.
- Impact Reporting: Recipients of funding are required to provide regular reports on the impact of their projects. This helps ensure that funds are being used effectively and that the DAO's goals are being met.
Remember, each step requires careful planning and execution to ensure the success of the program. It’s also important to comply with all relevant regulations and laws.
Development of Algorand Blockchain
Developing on Algorand blockchain involves using tools like AlgoSDK to interact with the blockchain. Developers can create smart contracts, handle transactions, and manage assets programmatically, enabling a wide range of decentralized applications.
To begin development, we need to install algosdk library and import algosdk, a crucial step in initializing our Algorand blockchain project. The example is as follows:
const algosdk = require("algosdk"); // Import AlgoSdk Library
We need to create an Algo Client using server, port and token.
const token = "YOUR TOKEN HERE";
const server = "https://testnet-api.algonode.cloud/";
const port = "PORT HERE";
// Algo Client creation
let algodClient = new algosdk.Algodv2(token, server, port);
Create an Account
To create a smart contract, first we need a creator account. To create an account on Algorand, we can utilize the generateAccount() method provided by AlgoSdk.
let creator = algosdk.generateAccount();
What are Smart Contracts
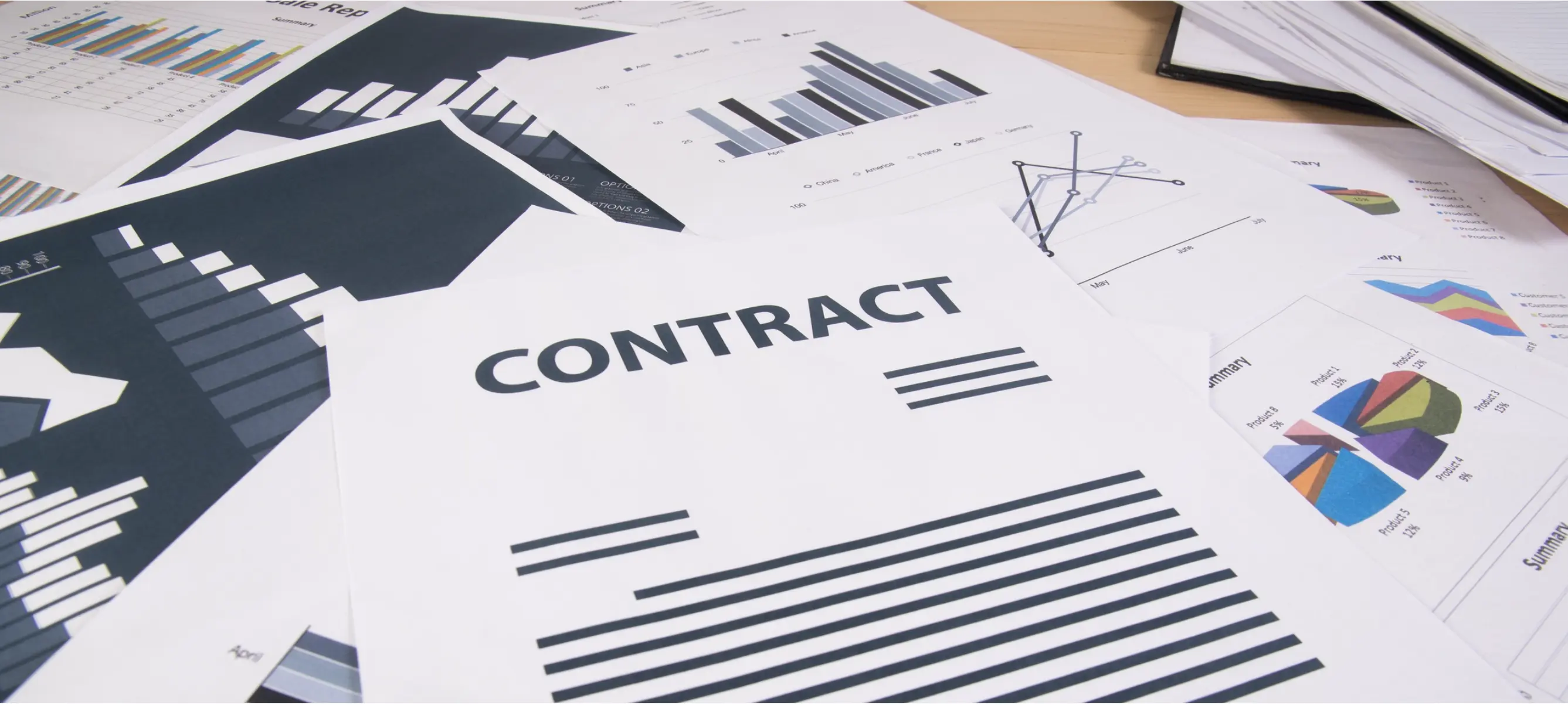
Smart contracts in Algorand are self-executing contracts with terms written in code, deployed on the Algorand blockchain. They automatically execute when conditions are met, enabling decentralized applications, asset management, and voting mechanisms. Algorand's efficient proof-of-stake consensus ensures fast transactions and low fees.
In summary, smart contracts are the backbone of DAOs, providing the automation and trustless execution that are essential for decentralized autonomous organizations to function effectively.
Smart Contract Storages
Smart contracts have 3 different types of storages
- Global Storage: Data that is stored on the blockchain for a contract globally. It can contain between 0 and 64 key/value pairs.
- Local Storage: Local storage in Algorand is the storage capacity of smart contracts that is specific to each account that has opted into one.
- Box Storage: Applications that need to maintain a large amount of state can use Box data storage.
Create a Smart Contract
Create a JavaScript function for deploying a smart contract on Algorand.
async function createDAO(){
// Smart Contract creation method
}
we first need to write the logic in Teal (Transaction Execution Approval Language) and then compile this Teal logic into a binary format that can be executed by the Algorand Virtual Machine (AVM). This compiled Teal code is what defines the behavior of the smart contract on the Algorand blockchain.
Prepare a Teal Logic
Teal (Transaction Execution Approval Language) is Algorand's efficient, secure, and deterministic smart contract language. It enables complex logic for various functionalities like multi-signature transactions. Teal is stack-based, compiled to a binary format, and executed by the Algorand Virtual Machine (AVM), ensuring consistent behavior across the network.
We need to create an approval program and also a clear program. Include these steps into the function called createDAO.
const approvalProgramSource = `#pragma version 2
txn ApplicationID
int 0
==
bnz main_l6
txn OnCompletion
int NoOp
==
bnz main_l3
err
main_l3:
txn Sender
byte "creator"
app_global_get
==
bnz main_l5
int 0
return
main_l5:
byte "vote_counter"
byte "vote_counter"
app_global_get
int 1
+
app_global_put
int 1
return
main_l6:
byte "creator"
txn Sender
app_global_put
int 1
return`;
const clearProgramSource = `#pragma version 2
int 1`;
Compile Teal source
After creating the Teal logic for the smart contract and the JavaScript method to deploy it, the next step is to compile both Teal sources. This involves using the Algo SDK's compileTeal function to convert the Teal code into a binary format that can be executed on the Algorand blockchain. This compiled Teal code is then used in the JavaScript method to deploy the smart contract.
//compile teal codes
const approvalCompileResp = await algodClient
.compile(Buffer.from(approvalProgramSource)).do();
const compiledApprovalProgram = new Uint8Array(
Buffer.from(approvalCompileResp.result, 'base64')
);
const clearCompileResp = await algodClient
.compile(Buffer.from(clearProgramSource)).do();
const compiledClearProgram = new Uint8Array(
Buffer.from(clearCompileResp.result, 'base64')
);
Define Global and Local storages
// define uint64s and byte slices stored in global/local storage
const numGlobalByteSlices = 1;
const numGlobalInts = 1;
const numLocalByteSlices = 1;
const numLocalInts = 1;
Deploy the Smart contract onto the Blockchain
Use the makeApplicationCreateTxnFromObject() method to deploy a smart contract onto the blockchain.
const suggestedParams = await algodClient.getTransactionParams().do();
// Create Smart contract
const appCreateTxn = algosdk.makeApplicationCreateTxnFromObject({
from: creatorAcc.addr,
approvalProgram: compiledApprovalProgram,
clearProgram: compiledClearProgram,
numGlobalByteSlices,
numGlobalInts,
numLocalByteSlices,
numLocalInts,
suggestedParams,
onComplete: algosdk.OnApplicationComplete.NoOpOC,
});
Sign in transaction and get AppId of smart contract
The App ID of a smart contract on the Algorand blockchain is a unique identifier assigned to the smart contract when it is created. This ID is used to reference the smart contract in subsequent transactions and interactions.
// Sign and send
await algodClient
.sendRawTransaction(appCreateTxn.signTxn(creatorAcc.sk))
.do();
const result = await algosdk.waitForConfirmation(
algodClient,
appCreateTxn.txID().toString(),
3
);
// Grab app id from confirmed transaction result
const appId = result['application-index'];
console.log(`Created app with index: ${appId}`);
Interact with a Smart contract
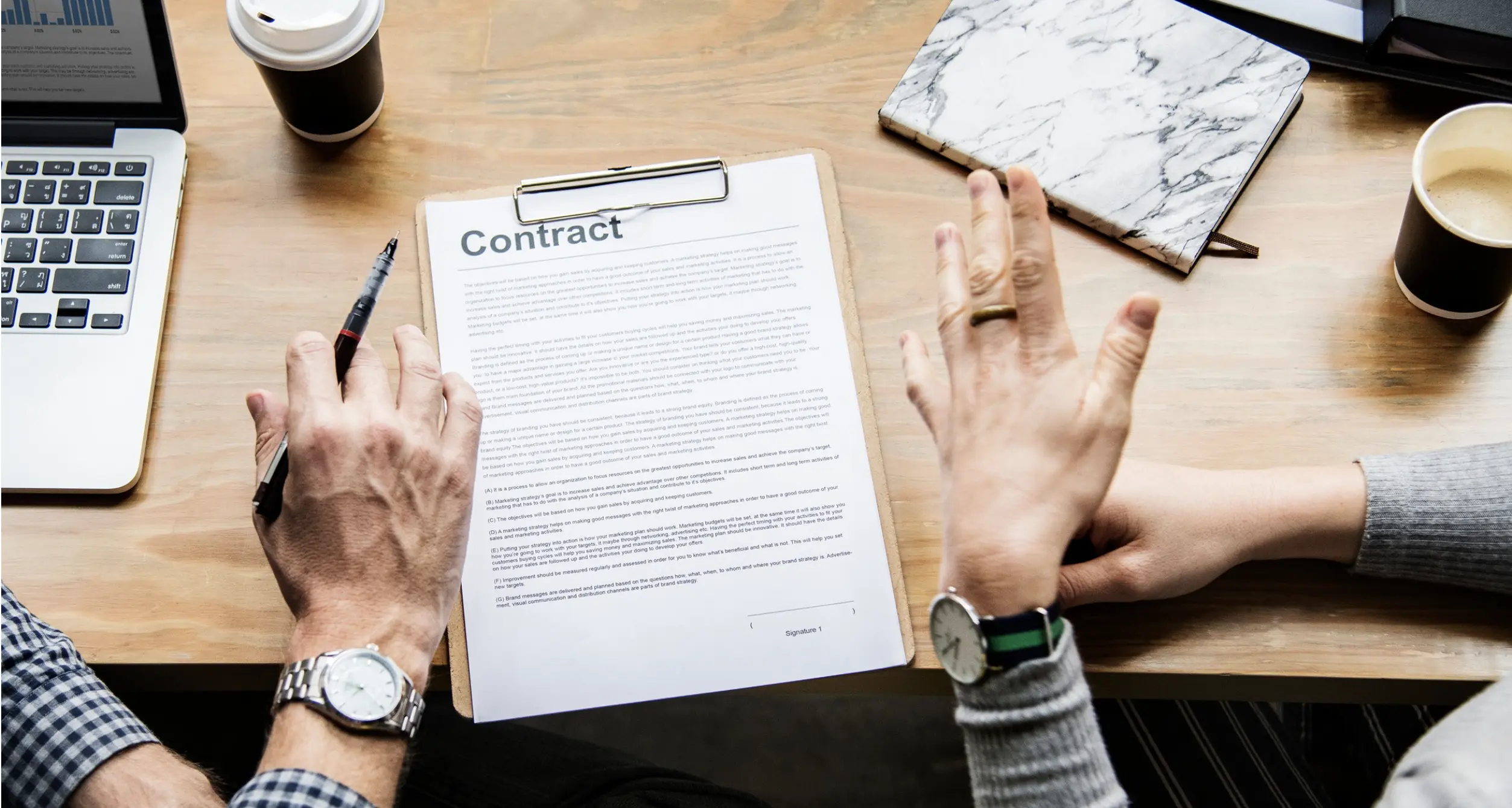
async function optIn(){
const suggestedParams = await algodClient.getTransactionParams().do();
// Opt in with user account
const appOptInTxn = algosdk.makeApplicationOptInTxnFromObject({
from: userAcc.addr,
appIndex: 640569588,
suggestedParams,
});
// Sign in transaction
await algodClient.sendRawTransaction(appOptInTxn.signTxn(userTwoAcc.sk)).do();
await algosdk.waitForConfirmation(algodClient, appOptInTxn.txID().toString(), 3);
}
async function callNoOp(){
const suggestedParams = await algodClient.getTransactionParams().do();
const appNoOpTxn = algosdk.makeApplicationNoOpTxnFromObject({
from: userAcc.addr,
appIndex: 640569588,
suggestedParams,
});
await algodClient.sendRawTransaction(appNoOpTxn.signTxn(userTwoAcc.sk)).do();
await algosdk.waitForConfirmation(algodClient, appNoOpTxn.txID().toString(), 3);
}
You can learn more about Algorand Development using the following link: https://developer.algorand.org/docs
#ceyentra #blockchain #algorand #charitablefunds